23 Jan2016
Association entre classe User et classe Profil
Objectif :
- Implémentation appropriée du principe de protection des données (encapsulation).
- Utilisation correcte des classes
- Respect des règles syntaxiques.
Travail à faire :
Soit le diagramme de classe suivant :
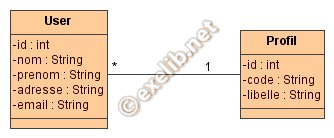
Figure 1 : Diagramme de classe
- Développer la classe User et la classe Profil dans le package « projet».
Chaque classe doit comporter :
- Un constructeur d’initialisation,
- La méthode toString,
- Développer une classe de test dans le package « projet.test».
Dans la classe de test créer :
- 3 profils:
- Chef de projet (CP),
- Manager (MN),
- Développeur (DP).
- 3 utilisateurs: Les deux premiers sont des managers et le dernier est un développeur.
- Afficher les informations de chaque utilisateur sous la forme :
L’email du manager ALAMI KARIM est k [DOT] alami [AT] gmail [DOT] com
Structure du projet :
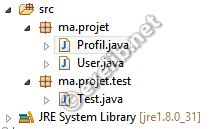
Structure du projet
Classe Profil :
package ma.projet; public class Profil { private int id; private String code; private String libelle; private static int comp; public Profil(String code, String libelle) { this.id = ++comp ; this.code = code; this.libelle = libelle; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getCode() { return code; } public void setCode(String code) { this.code = code; } public String getLibelle() { return libelle; } public void setLibelle(String libelle) { this.libelle = libelle; } @Override public String toString() { return id+" "+code+" "+libelle; } }
Classe User :
package ma.projet; public class User { private int id; private String nom; private String prenom; private String adresse; private String email; private Profil profil; private static int comp; public User(String nom, String prenom, String adresse, String email, Profil profil) { this.id = ++comp; this.nom = nom; this.prenom = prenom; this.adresse = adresse; this.email = email; this.profil = profil; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getNom() { return nom; } public void setNom(String nom) { this.nom = nom; } public String getPrenom() { return prenom; } public void setPrenom(String prenom) { this.prenom = prenom; } public String getAdresse() { return adresse; } public void setAdresse(String adresse) { this.adresse = adresse; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public Profil getProfil() { return profil; } public void setProfil(Profil profil) { this.profil = profil; } public String toString(){ return "Je suis le "+this.profil.getLibelle()+ " "+this.nom+" "+this.prenom+" mon email est : "+this.email; } }
Classe Test :
package ma.projet.test; import ma.projet.Profil; import ma.projet.User; public class Test { public static void main(String[] args) { Profil profils[] = new Profil[3]; profils[0] = new Profil("MN", "Manager"); profils[1] = new Profil("CP", "Chef de projet"); profils[2] = new Profil("DP", "Developpeur"); User users[] = new User[2]; users[0] = new User("Safi", "Amal", "Rabat", "a [DOT] safi [AT] gmail [DOT] com", profils[0]); users[1] = new User("Rami", "Ali", "Casa", "a [DOT] rami [AT] gmail [DOT] com", profils[2]); for (User u : users) { System.out.println(u); } } }
Sélectionnez le fichier à afficher.
|