19 Avr2016
Gestion des clients avec JDBC-Statement
Objectifs :
- Se connecter à une base de données avec JAVA.
- Développement des crud dans une application JAVA.
- Découvrir l'API JDBC.
Énoncé :
On souhaite créer une simple application pour la gestion des clients. Pour ce faire, on propose la classe Client caractérisée par : id, nom et le prénom.
Développement de la couche métier
- Créer la classe client dans le package "ma.projet.beans".
Développement de la couche de données
- Créer la table client dans une base de données nommée "demoJDBC".
Développement de la couche accès aux données
- Créer la classe Connexion dans le package "ma.projet.connexion"
- Créer une interfaces IDao dans le package "ma.projet.dao", cette interface contient les méthodes :
- boolean create ( T o) : Méthode permettant d'ajouter un objet o de type T.
- boolean delete (T o) : Méthode permettant de supprimer un objet o de type T.
- boolean update (T o) : Méthode permettant de modifier un objet o de type T.
- T findById (int id) : Méthode permettant de renvoyer un objet dont id est passé en paramètre.
- List <T> findAll ( ) : Méthode permettant de renvoyer la liste des objets de type T.
- boolean create ( T o) : Méthode permettant d'ajouter un objet o de type T.
- Créer la classe ClientService qui implémente l'interface IDao dans le package "ma.projet.service" et redéfinir toutes les méthodes de l'interfaces.
Développement de la couche présentation
- Dans une classe de test :
- Créer 5 clients.
- Afficher le client dont id = 3.
- Supprimer le client dont id = 3.
- Modifier le client dont id = 2.
- Afficher la liste des clients.
Structure de projet
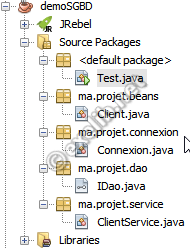
Structure de projet
La classe Client :
package ma.projet.beans; /** * * @author lachgar */ public class Client { private int id; private String nom; private String prenom; public Client(String nom, String prenom) { this.nom = nom; this.prenom = prenom; } public Client(int id, String nom, String prenom) { this.id = id; this.nom = nom; this.prenom = prenom; } public int getId() { return id; } public String getNom() { return nom; } public void setNom(String nom) { this.nom = nom; } public String getPrenom() { return prenom; } public void setPrenom(String prenom) { this.prenom = prenom; } }
Le code SQL pour la création de la table Client :
-- -- Structure de la table `client` -- CREATE TABLE IF NOT EXISTS `client` ( `id` int(11) NOT NULL AUTO_INCREMENT, `nom` varchar(50) NOT NULL, `prenom` varchar(50) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=14 ;
La classe Connexion :
package ma.projet.connexion; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; /** * * @author lachgar */ public class Connexion { private static String login = "root"; private static String password = ""; private static String url = "jdbc:mysql://localhost/demoJDBC"; private static Connection cn; static { try { //Étape 1 : Charger le driver de la base de données // cible Class.forName("com.mysql.jdbc.Driver"); //Étape 2 : Authentification auprès de la base de données // et sélectionner le schéma cn = DriverManager.getConnection(url, login, password); } catch (ClassNotFoundException ex) { System.out.println("Impossible de charger le driver"); } catch (SQLException ex) { System.out.println("Erreur de connexion"); } } public static Connection getCn() { return cn; } }
L'interface IDao :
package ma.projet.dao; import java.util.List; /** * * @author lachgar */ public interface IDao< T> { boolean create(T o); boolean update(T o); boolean delete(T o); T findById(int id); List<T> findAll(); }
La classe ClientService :
package ma.projet.service; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.List; import ma.projet.beans.Client; import ma.projet.connexion.Connexion; import ma.projet.dao.IDao; /** * * @author lachgar */ public class ClientService implements IDao<Client> { @Override public boolean create(Client o) { try { //Création d'une requête SQL String req = "insert into client (nom, prenom) values ('" + o.getNom() + "', '" + o.getPrenom() + "')"; //Étape 3 : Création d'un Stalement Statement st = Connexion.getCn().createStatement(); //Étape 4 : Exécution de la requête if (st.executeUpdate(req) == 1) { return true; } } catch (SQLException ex) { System.out.println("Erreur SQL"); } return false; } @Override public boolean update(Client o) { try { String req = "update client set nom ='"+o.getNom()+"', prenom ='"+o.getPrenom()+"' where id = "+o.getId(); Statement st = Connexion.getCn().createStatement(); if(st.executeUpdate(req) == 1) return true; } catch (SQLException ex) { System.out.println("Erreur SQL"); } return false; } @Override public boolean delete(Client o) { try { String req = "delete from client where id=" + o.getId(); Statement st = Connexion.getCn().createStatement(); if (st.executeUpdate(req) == 1) { return true; } } catch (SQLException ex) { System.out.println("Erreur SQL"); } return false; } @Override public Client findById(int id) { try { String req = "select * from client where id=" + id; Statement st = Connexion.getCn().createStatement(); ResultSet rs = st.executeQuery(req); if (rs.next()) { return new Client(rs.getInt("Id"), rs.getString("nom"), rs.getString("prenom")); } } catch (SQLException ex) { System.out.println("Erreur SQL"); } return null; } @Override public List<Client> findAll() { List<Client> clients = new ArrayList<>(); try { String req = "select * from client"; Statement st = Connexion.getCn().createStatement(); ResultSet rs = st.executeQuery(req); while (rs.next()) { clients.add(new Client(rs.getInt("Id"), rs.getString("nom"), rs.getString("prenom"))); } } catch (SQLException ex) { System.out.println("Erreur SQL"); } return clients; } }
La classe de Test :
import ma.projet.beans.Client; import ma.projet.service.ClientService; /** * * @author lachgar */ public class Test { public static void main(String[] args) { ClientService cs = new ClientService(); //Création des clients cs.create(new Client("SAFI", "ali")); cs.create(new Client("ALAOUI", "widane")); cs.create(new Client("RAMI", "amine")); cs.create(new Client("ALAMI", "kamal")); cs.create(new Client("SELAMI", "mohamed")); //Afficher le client dont id = 3 Client c = cs.findById(3); System.out.println(c.getNom()+" "+c.getPrenom()); //Supprimer le client dont id = 3 cs.delete(cs.findById(3)); //Modifier le client dont id = 2 Client cc = cs.findById(2); cc.setNom("nouveau nom"); cc.setPrenom("nouveau Prénom"); cs.update(c); //Afficher la liste des clients for(Client cl : cs.findAll()) System.out.println(cl.getNom()); } }