16 Fév2016
Gestion des clients avec ADO.net
Objectifs:
- Accéder aux données à l’aide de l’ADO.NET
- Associer les contrôles DropDownList et GridView avec une source de données
Énoncé:
1) Créer une page ASP.NET qui permet la gestion d'une table Client avec les opérations d’ajout, de suppression et de modification en utilisant le mode connecté du Framework ADO.NET.
Un client est caractérisé par son identifiant (auto-incrémenté), son nom, son prénom, son adresse et sa ville.
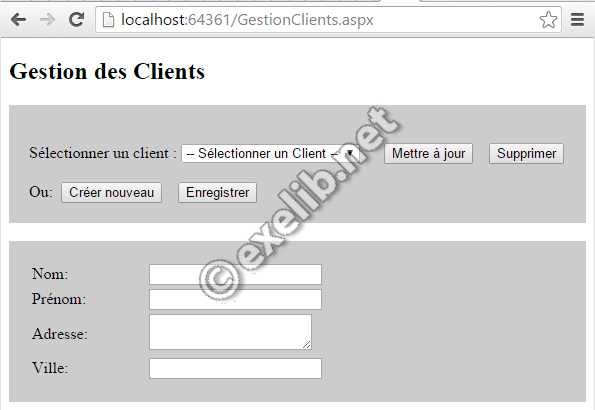
Interface de la gestion des clients
L'interface doit contenir les éléments suivants:
- une liste pré-remplit des noms et prénoms des clients (séparés par un espace)
- si un client est sélectionné ses informations doivent apparaître dans les zones de texte
- un bouton "Mettre à jour" qui permet de valider les modifications effectuées dans les zones de texte
- un bouton "Supprimer" qui permet la suppression du client sélectionné
- un bouton "Créer nouveau" qui vide les zones de texte et donne le focus au champ "Nom"
- un bouton "Enregistrer" qui insère un nouveau client
2) Créer une page de consultation qui permet l'affichage de la liste des clients avec filtrage par ville.
Page de gestion des clients
Code ASP.net
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="GestionClients.aspx.cs" Inherits="tp5_exelib.GestionClients" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> <title></title> <style type="text/css"> .auto-style1 { width: 113px; } .auto-style2 { color: #006600; } </style> </head> <body> <form id="form1" runat="server"> <h2>Gestion des Clients</h2> <div style="background-color: #CCCCCC; padding:20px"> <br /> Sélectionner un client : <asp:DropDownList ID="drpClients" runat="server" OnSelectedIndexChanged="drpClients_SelectedIndexChanged"> </asp:DropDownList> <asp:Button ID="btnMiseAJour" runat="server" Text="Mettre à jour" OnClick="btnMiseAJour_Click" /> <asp:Button ID="btnSupprimer" runat="server" Text="Supprimer" OnClick="btnSupprimer_Click" /> <br /> <br /> Ou: <asp:Button ID="btnNouveau" runat="server" Text="Créer nouveau" OnClick="btnNouveau_Click" /> <asp:Button ID="btnEnregistrer" runat="server" Text="Enregistrer" OnClick="btnEnregistrer_Click" /> <br /> </div> <br /> <div style="background-color: #CCCCCC ; padding:20px"> <strong> <asp:Label ID="lblMsg" runat="server" Text="Label" CssClass="auto-style2"></asp:Label> </strong> <table> <tr> <td class="auto-style1">Nom:</td> <td><asp:TextBox ID="txtNom" runat="server"></asp:TextBox></td> </tr> <tr> <td class="auto-style1">Prénom:</td> <td><asp:TextBox ID="txtPrenom" runat="server"></asp:TextBox></td> </tr> <tr> <td class="auto-style1">Adresse:</td> <td><asp:TextBox ID="txtAdresse" runat="server" TextMode="MultiLine"></asp:TextBox></td> </tr> <tr> <td class="auto-style1">Ville:</td> <td><asp:TextBox ID="txtVille" runat="server"></asp:TextBox></td> </tr> </table> </div> </form> </body> </html>
Code C#
namespace tp5_exelib { public partial class GestionClients : System.Web.UI.Page { static string ch = ConfigurationManager.ConnectionStrings["gestionCmd"].ConnectionString; //Procédure qui remplit le DropDownList avec la liste des clients protected void RemplirListeClients() { drpClients.Items.Clear(); SqlConnection con = new SqlConnection(ch); string sql = "SELECT id, Nom + ' ' + Prenom as NomPrenom FROM Clients Order By NomPrenom"; SqlCommand cmd = new SqlCommand(sql, con); try { con.Open(); drpClients.DataSource = cmd.ExecuteReader(); drpClients.DataTextField = "NomPrenom"; drpClients.DataValueField = "id"; drpClients.DataBind(); drpClients.Items.Insert(0, "-- Sélectionner un Client --"); } finally { con.Close(); } } //Procédure qui vide les zones de texte protected void ViderTextBox() { txtNom.Text = ""; txtPrenom.Text = ""; txtAdresse.Text = ""; txtVille.Text = ""; txtNom.Focus(); } protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { drpClients.AutoPostBack = true; RemplirListeClients(); } lblMsg.Text = ""; } protected void drpClients_SelectedIndexChanged(object sender, EventArgs e) { if(drpClients.SelectedIndex > 0) { SqlConnection con = new SqlConnection(ch); string req = "SELECT * FROM Clients WHERE id=@id"; SqlCommand cmd = new SqlCommand(req, con); cmd.Parameters.AddWithValue("@id", drpClients.SelectedValue); SqlDataReader dr; try { con.Open(); dr = cmd.ExecuteReader(); if (dr.Read()) { txtNom.Text = dr["Nom"].ToString(); txtPrenom.Text = dr["Prenom"].ToString(); txtAdresse.Text = dr["Adresse"].ToString(); txtVille.Text = dr["Ville"].ToString(); } dr.Close(); } finally { con.Close(); } } else { ViderTextBox(); } } protected void btnMiseAJour_Click(object sender, EventArgs e) { //Tester si un client est sélectionné if (drpClients.SelectedIndex > 0) { string sql = "UPDATE Clients SET Nom=@nom, Prenom=@prenom, Adresse=@adr, Ville=@ville WHERE Id=@id"; SqlConnection con = new SqlConnection(ch); SqlCommand cmd = new SqlCommand(sql, con); cmd.Parameters.AddWithValue("@id", drpClients.SelectedValue); cmd.Parameters.AddWithValue("@nom", txtNom.Text); cmd.Parameters.AddWithValue("@prenom", txtPrenom.Text); cmd.Parameters.AddWithValue("@adr", txtAdresse.Text); cmd.Parameters.AddWithValue("@ville", txtVille.Text); int modification = 0; try { con.Open(); modification = cmd.ExecuteNonQuery(); } finally { con.Close(); } //Actualiser la liste des clients après modification if (modification > 0) { RemplirListeClients(); lblMsg.Text = "Modification effectuée avec succès"; } } else { lblMsg.Text = "Veuillez sélectionner un client!"; } } protected void btnSupprimer_Click(object sender, EventArgs e) { //Tester si un client est sélectionné if (drpClients.SelectedIndex > 0) { string sql = "DELETE FROM Clients WHERE Id=@id"; SqlConnection con = new SqlConnection(ch); SqlCommand cmd = new SqlCommand(sql, con); cmd.Parameters.AddWithValue("@id", drpClients.SelectedValue); int suppression = 0; try { con.Open(); suppression = cmd.ExecuteNonQuery(); } finally { con.Close(); } //Vider les zones de texte et actualiser la liste des clients après suppression if (suppression > 0) { ViderTextBox(); RemplirListeClients(); lblMsg.Text = "Client supprimé avec succès"; } } else { lblMsg.Text = "Veuillez sélectionner un client!"; } } protected void btnNouveau_Click(object sender, EventArgs e) { ViderTextBox(); } protected void btnEnregistrer_Click(object sender, EventArgs e) { string sql = "INSERT INTO Clients(Nom, Prenom, Adresse, Ville) VALUES(@nom,@prenom,@adr,@ville)"; SqlConnection con = new SqlConnection(ch); SqlCommand cmd = new SqlCommand(sql, con); cmd.Parameters.AddWithValue("@nom", txtNom.Text); cmd.Parameters.AddWithValue("@prenom", txtPrenom.Text); cmd.Parameters.AddWithValue("@adr", txtAdresse.Text); cmd.Parameters.AddWithValue("@ville", txtVille.Text); int ajout = 0; try { con.Open(); ajout = cmd.ExecuteNonQuery(); } finally { con.Close(); } //Vider les zones de texte et actualiser la liste des clients après ajout if (ajout > 0) { ViderTextBox(); RemplirListeClients(); lblMsg.Text = "Client ajouté avec succès"; } } } }
Page de consultation et filtrage des clients
Code ASP.net
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="ListeClientsVille.aspx.cs" Inherits="tp5_exelib.ListeClientsVille" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Button ID="Button1" runat="server" OnClick="Button1_Click" Text="Afficher tous les clients" /> <br /> <br /> <asp:Label ID="Label1" runat="server" Text="Filtrer par ville: "></asp:Label> <asp:DropDownList ID="drpVille" runat="server" OnSelectedIndexChanged="drpVille_SelectedIndexChanged"> </asp:DropDownList> <br /> <br /> <asp:GridView ID="GridView1" runat="server" CellPadding="4" ForeColor="#333333" GridLines="None"> <AlternatingRowStyle BackColor="White" ForeColor="#284775" /> <EditRowStyle BackColor="#999999" /> <FooterStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" /> <HeaderStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" /> <PagerStyle BackColor="#284775" ForeColor="White" HorizontalAlign="Center" /> <RowStyle BackColor="#F7F6F3" ForeColor="#333333" /> <SelectedRowStyle BackColor="#E2DED6" Font-Bold="True" ForeColor="#333333" /> <SortedAscendingCellStyle BackColor="#E9E7E2" /> <SortedAscendingHeaderStyle BackColor="#506C8C" /> <SortedDescendingCellStyle BackColor="#FFFDF8" /> <SortedDescendingHeaderStyle BackColor="#6F8DAE" /> </asp:GridView> </div> </form> </body> </html>
Code C#
namespace tp5_exelib { public partial class ListeClientsVille : System.Web.UI.Page { static string ch = ConfigurationManager.ConnectionStrings["gestionCmd"].ConnectionString; protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { drpVille.AutoPostBack = true; SqlConnection con = new SqlConnection(ch); SqlCommand cmd = new SqlCommand("SELECT DISTINCT Ville FROM Clients", con); try { con.Open(); drpVille.DataSource = cmd.ExecuteReader(); drpVille.DataTextField = "Ville"; drpVille.DataBind(); drpVille.Items.Insert(0, "--Sélectionner une ville--"); } finally { con.Close(); } } } protected void Button1_Click(object sender, EventArgs e) { SqlConnection con = new SqlConnection(ch); SqlCommand cmd = new SqlCommand("SELECT * FROM Clients", con); try { con.Open(); GridView1.DataSource = cmd.ExecuteReader(); GridView1.DataBind(); } finally { con.Close(); } } protected void drpVille_SelectedIndexChanged(object sender, EventArgs e) { SqlConnection con = new SqlConnection(ch); string sql = "SELECT * FROM Clients WHERE ville=@ville"; SqlCommand cmd = new SqlCommand(sql, con); cmd.Parameters.AddWithValue("@ville", drpVille.SelectedItem.Value); try { con.Open(); GridView1.DataSource = cmd.ExecuteReader(); GridView1.DataBind(); } finally { con.Close(); } } } }
La chaîne de connexion à paramétrer et ajouter dans le fichier Web.config
<connectionStrings> <add name="gestionCmd" connectionString="server=localhost\sqlexpress;database=gestionCmd;Integrated Security=True" providerName="System.Data.SqlClient" /> </connectionStrings>