Affichage du calendrier des matchs d’un championnat
Objectifs:
- Comprendre le cycle de vie des événements d’une page ASP.net
- Utiliser la propriété isPostBack
- Manipuler les contrôles DropDownList et Calendar
Énoncé:
L’objectif de cet exercice est de réaliser une page web qui permet la consultation et la modification des dates des matchs d’un championnat en utilisant un calendrier.
1) Créer la page web suivante qui contient un contrôle DropDownList listMatch pré-remplie avec une liste des matchs, un contrôle Calendar calDate et un contrôle Label lblMatchs pour afficher la date du match sélectionné :
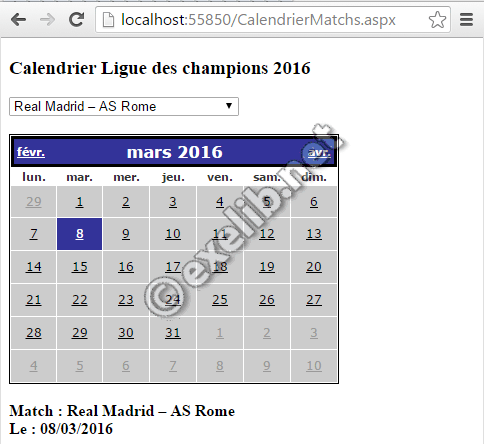
Lorsque l’utilisateur sélectionne un match sa date sera sélectionnée dans le calendrier et sera affiché dans le Label.
Note : Le calendrier sera caché si aucun match n’est sélectionné.
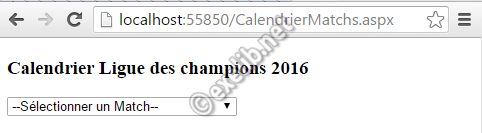
Un exemple des dates des 10 premiers matchs de la Ligue des champions 2016 :
- 16/02/2016: PSG – Chelsea
- 16/02/2016: Benfica – Zénith Saint-Pétersbourg
- 17/02/2016: La Gantoise – Wolfsburg
- 17/02/2016: AS Rome – Real Madrid
- 23/02/2016: Arsenal – Barcelone
- 23/02/2016: Juventus – Bayern Munich
- 24/02/2016: PSV Eindhoven – Atletico Madrid
- 24/02/2016: Dynamo Kiev– Manchester City
- 08/03/2016: Wolfsburg – La Gantoise
- 08/03/2016: Real Madrid – AS Rome
2) Modifier l’interface pour permettre aussi l’affichage de tous les matchs programmés dans la même date qu’on sélectionne dans le calendrier. L’affichage sera effectué dans un Label et devra prévoir le cas où aucun match n’est programmé :
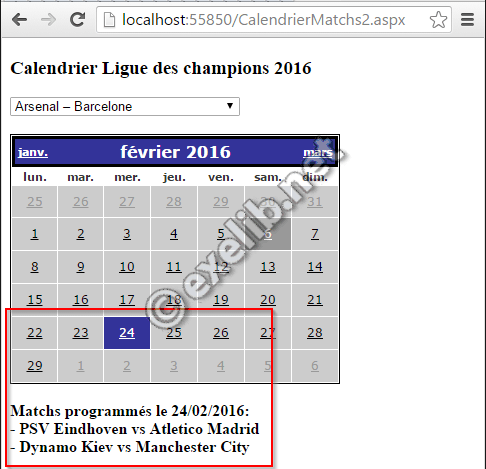
Cas : Aucun match programmé
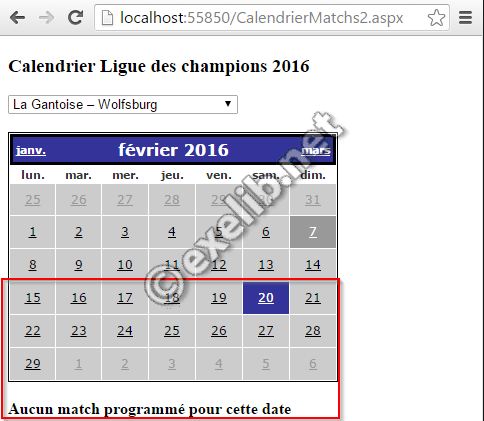
- Permettre la modification de la date du match sélectionné
- Afficher les drapeaux des équipes sélectionnées
Question 1 - Solution 1:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="CalendrierMatchs.aspx.cs" Inherits="tp2_exelib.CalendrierMatchs" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> <title></title> </head> <body> <form id="form1" runat="server"> <h3>Calendrier Ligue des champions 2016</h3> <div> <asp:DropDownList ID="listMatch" runat="server" AutoPostBack="True" OnSelectedIndexChanged="listMatch_SelectedIndexChanged"> </asp:DropDownList> <br /> <br /> <asp:Calendar ID="calDate" runat="server" BackColor="White" BorderColor="Black" BorderStyle="Solid" CellSpacing="1" Font-Names="Verdana" Font-Size="9pt" ForeColor="Black" Height="250px" NextPrevFormat="ShortMonth" Width="330px"> <DayHeaderStyle Font-Bold="True" Font-Size="8pt" ForeColor="#333333" Height="8pt" /> <DayStyle BackColor="#CCCCCC" /> <NextPrevStyle Font-Bold="True" Font-Size="8pt" ForeColor="White" /> <OtherMonthDayStyle ForeColor="#999999" /> <SelectedDayStyle BackColor="#333399" ForeColor="White" /> <TitleStyle BackColor="#333399" BorderStyle="Solid" Font-Bold="True" Font-Size="12pt" ForeColor="White" Height="12pt" /> <TodayDayStyle BackColor="#999999" ForeColor="White" /> </asp:Calendar> <br /> <asp:Label ID="lblMatchs" runat="server" style="font-weight: 700"></asp:Label> </div> </form> </body> </html>
public partial class CalendrierMatchs : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { listMatch.Items.Add("--Sélectionner un Match--"); listMatch.Items.Add("PSG – Chelsea"); listMatch.Items.Add("Benfica – Zénith Saint-Pétersbourg"); listMatch.Items.Add("La Gantoise – Wolfsburg"); listMatch.Items.Add("AS Rome – Real Madrid"); listMatch.Items.Add("Arsenal – Barcelone"); listMatch.Items.Add("Juventus – Bayern Munich"); listMatch.Items.Add("PSV Eindhoven – Atletico Madrid"); listMatch.Items.Add("Dynamo Kiev– Manchester City"); listMatch.Items.Add("Wolfsburg – La Gantoise"); listMatch.Items.Add("Real Madrid – AS Rome"); calDate.Visible = false; } } protected void listMatch_SelectedIndexChanged(object sender, EventArgs e) { calDate.Visible = true; switch (listMatch.SelectedIndex) { case 1: calDate.SelectedDate = new DateTime(2016, 02, 16); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 2: calDate.SelectedDate = new DateTime(2016, 02, 16); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 3: calDate.SelectedDate = new DateTime(2016, 02, 17); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 4: calDate.SelectedDate = new DateTime(2016, 02, 17); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 5: calDate.SelectedDate = new DateTime(2016, 02, 23); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 6: calDate.SelectedDate = new DateTime(2016, 02, 23); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 7: calDate.SelectedDate = new DateTime(2016, 02, 24); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 8: calDate.SelectedDate = new DateTime(2016, 02, 24); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 9: calDate.SelectedDate = new DateTime(2016, 03, 08); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; case 10: calDate.SelectedDate = new DateTime(2016, 03, 08); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.Text + "<br>Le : " + calDate.SelectedDate.ToShortDateString(); break; default: calDate.Visible = false; lblMatchs.Text = ""; break; } } }
Question 2 - Solution 1:
protected void calDate_SelectionChanged(object sender, EventArgs e) { switch (calDate.SelectedDate.ToShortDateString()) { case "16/02/2016": lblMatchs.Text = "Matchs programmés le 16/02/2016:<br>"; lblMatchs.Text += "- PSG vs Chelsea<br>- Benfica vs Zénith Saint-Pétersbourg"; break; case "17/02/2016": lblMatchs.Text = "Matchs programmés le 17/02/2016:<br>"; lblMatchs.Text += "- La Gantoise vs Wolfsburg<br>- AS Rome vs Real Madrid"; break; case "23/02/2016": lblMatchs.Text = "Matchs programmés le 23/02/2016:<br>"; lblMatchs.Text += "- Arsenal vs Barcelone<br>- Juventus vs Bayern Munich"; break; case "24/02/2016": lblMatchs.Text = "Matchs programmés le 24/02/2016:<br>"; lblMatchs.Text += "- PSV Eindhoven vs Atletico Madrid<br>- Dynamo Kiev vs Manchester City"; break; case "08/03/2016": lblMatchs.Text = "Matchs programmés le 08/03/2016:<br>"; lblMatchs.Text += "- Wolfsburg vs La Gantoise<br>- Real Madrid vs AS Rome"; break; default: lblMatchs.Text = "Aucun match programmé pour cette date"; break; } }
Comme première solution n'est pas pratique surtout si on a plusieurs matchs dans la liste
Une autre solution consiste à utiliser la collection ListItem du contrôle DropDownList en remplissant la liste avec les matchs et leurs dates, ce qui permettra de récupérer facilement la date du match sélectionné.
Solution 2 avec ListItem:
public partial class CalendrierMatchsSolution2 : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { listMatch.Items.Add("--Sélectionner un Match--"); listMatch.Items.Add(new ListItem("PSG – Chelsea", "16/02/2016")); listMatch.Items.Add(new ListItem("Benfica – Zénith Saint-Pétersbourg", "16/02/2016")); listMatch.Items.Add(new ListItem("La Gantoise – Wolfsburg", "17/02/2016")); listMatch.Items.Add(new ListItem("AS Rome – Real Madrid", "17/02/2016")); listMatch.Items.Add(new ListItem("Arsenal – Barcelone", "23/02/2016")); listMatch.Items.Add(new ListItem("Juventus – Bayern Munich", "23/02/2016")); listMatch.Items.Add(new ListItem("PSV Eindhoven – Atletico Madrid", "24/02/2016")); listMatch.Items.Add(new ListItem("Dynamo Kiev– Manchester City", "24/02/2016")); listMatch.Items.Add(new ListItem("Wolfsburg – La Gantoise", "08/03/2016")); listMatch.Items.Add(new ListItem("Real Madrid – AS Rome", "08/03/2016")); calDate.Visible = false; } } protected void listMatch_SelectedIndexChanged(object sender, EventArgs e) { if(listMatch.SelectedIndex < 1) { calDate.Visible = false; lblMatchs.Text = ""; } else { calDate.Visible = true; string dateMatch = listMatch.SelectedValue; calDate.SelectedDate = Convert.ToDateTime(dateMatch); calDate.VisibleDate = calDate.SelectedDate; lblMatchs.Text = "Match : " + listMatch.SelectedItem.Text + "<br>Le : " + dateMatch; } } protected void calDate_SelectionChanged(object sender, EventArgs e) { string strListMatchs = ""; foreach(ListItem item in listMatch.Items) { if(item.Value == calDate.SelectedDate.ToShortDateString()) { strListMatchs += "- " + item.Text + "<br>"; } } if(strListMatchs == "") lblMatchs.Text = "Aucun match programmé pour cette date"; else { lblMatchs.Text = "Matchs programmés le "+ calDate.SelectedDate.ToShortDateString() + ":<br>"; lblMatchs.Text += strListMatchs; } } }